Mixed Precision vs Standard Precision in AI Training
As deep learning models grow in complexity and size, the demand for efficient training techniques becomes increasingly important. One area that significantly impacts training performance is numerical precision—the format used to represent floating-point numbers during computation. This blog explores the key differences between mixed and standard precision in AI training and when to use each for optimal results.
What is Precision in AI Training?
In AI training, precision refers to the number of bits representing floating-point numbers. The most commonly used formats include FP32 (32-bit floating point), FP16 (16-bit floating point), and BF16 (brain floating point 16-bit). Precision affects how data is stored, how fast operations are performed, and how accurately models can learn from data. Learn more about Floating Point Precision here.
The training of neural networks involves large matrix multiplications and gradient calculations. These operations are sensitive to the precision format used, as lower precision may introduce rounding errors or loss of detail, potentially impacting model convergence and final accuracy.
Standard Precision: FP32
FP32, or single-precision floating point, has long been the standard for training deep learning models. It offers a balanced mix of range and accuracy, making it suitable for a wide variety of training tasks.
The advantages of FP32 include:
- High numerical stability, especially for deep or sensitive models
- Better support for complex training operations without custom handling
However, the main drawbacks are higher memory usage and lower computational throughput, which can limit training speed and resource efficiency, especially for large-scale models.
Mixed Precision: Combining FP16/BF16 and FP32
Mixed precision training uses a combination of low-precision formats (such as FP16 or BF16) for most operations while retaining FP32 for critical parts like weight updates and loss accumulation. This hybrid approach leverages the performance benefits of low precision without compromising accuracy.
Modern GPUs, such as NVIDIA’s Tensor Core–enabled models, are optimized for mixed precision and can accelerate training significantly.
Key benefits include:
- Reduced memory consumption, allowing for larger batch sizes or deeper models
- Faster training throughput, due to increased parallelism and lower data movement
- Lower energy usage, contributing to cost-effective and sustainable training
Accuracy and Stability Considerations
Mixed precision is not without its challenges. The reduced numerical range of FP16 can cause underflows or overflows in certain computations. To mitigate this, training frameworks apply techniques such as loss scaling, which adjusts the scale of gradients to preserve detail during backpropagation.
In practice, most modern deep learning libraries like PyTorch and TensorFlow include automatic mixed precision (AMP) features that handle these adjustments seamlessly. While mixed precision generally maintains model accuracy, it may still fall short for niche models that are highly sensitive to numerical variance.
When to Use Each
Mixed precision is recommended in the following scenarios:
- Training large models such as transformers or CNNs
- Using GPUs with specialized hardware for FP16/BF16 operations
- Seeking to reduce training time or memory consumption
Standard precision (FP32) remains preferred when:
- Debugging or experimenting with new architectures
- Working with models prone to instability
- Targeting hardware without optimized mixed precision support
Enabling Mixed Precision Training (Quick Setup)
You can enable mixed precision in your training workflow with just one line of code in most frameworks:
Mixed Precision in PyTorch (with AMP)
PyTorch AMP or automatic mixed precision support is as easy as importing and wrapping the forward pass in autocast() and using GradScaler() for loss scaling. Add this to your training script:
import torch
from torch import nn, optim
from torch.cuda.amp import autocast, GradScaler
model = ##Your model architecture
optimizer = ##Your optimizer and learning rate
scaler = GradScaler()
for input, target in ##Your Data Loader :
optimizer.zero_grad()
with autocast():
output = model(input.cuda())
loss = loss_fn(##Your Loss Function
scaler.scale(loss).backward()
scaler.step(optimizer)
scaler.update()
You can set autocast(dtype=torch.bfloat16): in to change the precision to BF16.
If you're already using or considering a higher-level wrapper like PyTorch Lightning, you can enable mixed precision training very easily with this global command:
trainer = pl.Trainer(precision=16)
## For BF16 use: trainer = pl.Trainer(amp_dtype=torch.bfloat16)
Mixed Precision TensorFlow
Enable mixed precision globally and that's it—TensorFlow will automatically optimize supported layers with:
from tensorflow.keras import mixed_precision
mixed_precision.set_global_policy('mixed_float16')
You can set the global policy to BF16 with mixed_precision.set_global_policy('mixed_bfloat16'). TensorFlow automatically handles loss scaling and casting, and activates FP16 or BF16 to compatible layers.
Conclusion
Precision format plays a crucial role in AI training performance and accuracy. While standard FP32 offers stability and simplicity, mixed precision provides significant advantages in speed and resource efficiency when supported by modern hardware. For most production workloads, mixed precision is the preferred approach, delivering faster training without sacrificing model quality. However, FP32 remains a reliable choice in scenarios where numerical accuracy is ideal and necessary.
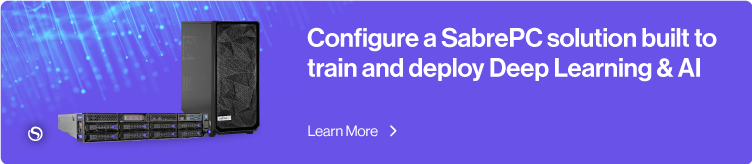